mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2024-11-15 19:38:26 +00:00
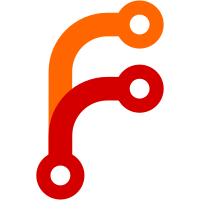
- Dispatcher sets a Controller::here variable with the real URL used to access the page, so that tag generators can that use an url (linkTo and formTag for example) use the real url, not guess it from the controller and action names which often fails - Log class works more reliably and a LogError() shortcut function was added - Nstring class added, to store string-related functions (there are just four yet, including a random password generator and an string-to-array splitter - SimpleTest library (with Rephlux) included in /vendors; I've tweaked SimpleScorer::inCli() function, because it didn't work on my setup, it should work everywhere now (it checks for empty REQUEST_METHOD, which should only be empty in CLI) git-svn-id: https://svn.cakephp.org/repo/trunk/cake@248 3807eeeb-6ff5-0310-8944-8be069107fe0
115 lines
No EOL
3.7 KiB
PHP
115 lines
No EOL
3.7 KiB
PHP
<?php
|
|
/**
|
|
* base include file for SimpleTest
|
|
* @package SimpleTest
|
|
* @subpackage UnitTester
|
|
* @version $Id$
|
|
*/
|
|
|
|
/**#@+
|
|
* include other SimpleTest class files
|
|
*/
|
|
require_once(dirname(__FILE__) . '/browser.php');
|
|
require_once(dirname(__FILE__) . '/xml.php');
|
|
require_once(dirname(__FILE__) . '/simple_test.php');
|
|
/**#@-*/
|
|
|
|
/**
|
|
* Runs an XML formated test on a remote server.
|
|
* @package SimpleTest
|
|
* @subpackage UnitTester
|
|
*/
|
|
class RemoteTestCase {
|
|
var $_url;
|
|
var $_dry_url;
|
|
var $_size;
|
|
|
|
/**
|
|
* Sets the location of the remote test.
|
|
* @param string $url Test location.
|
|
* @param string $dry_url Location for dry run.
|
|
* @access public
|
|
*/
|
|
function RemoteTestCase($url, $dry_url = false) {
|
|
$this->_url = $url;
|
|
$this->_dry_url = $dry_url ? $dry_url : $url;
|
|
$this->_size = false;
|
|
}
|
|
|
|
/**
|
|
* Accessor for the test name for subclasses.
|
|
* @return string Name of the test.
|
|
* @access public
|
|
*/
|
|
function getLabel() {
|
|
return $this->_url;
|
|
}
|
|
|
|
/**
|
|
* Runs the top level test for this class. Currently
|
|
* reads the data as a single chunk. I'll fix this
|
|
* once I have added iteration to the browser.
|
|
* @param SimpleReporter $reporter Target of test results.
|
|
* @returns boolean True if no failures.
|
|
* @access public
|
|
*/
|
|
function run(&$reporter) {
|
|
$browser = &$this->_createBrowser();
|
|
$xml = $browser->get($this->_url);
|
|
if (! $xml) {
|
|
trigger_error('Cannot read remote test URL [' . $this->_url . ']');
|
|
return false;
|
|
}
|
|
$parser = &$this->_createParser($reporter);
|
|
if (! $parser->parse($xml)) {
|
|
trigger_error('Cannot parse incoming XML from [' . $this->_url . ']');
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Creates a new web browser object for fetching
|
|
* the XML report.
|
|
* @return SimpleBrowser New browser.
|
|
* @access protected
|
|
*/
|
|
function &_createBrowser() {
|
|
return new SimpleBrowser();
|
|
}
|
|
|
|
/**
|
|
* Creates the XML parser.
|
|
* @param SimpleReporter $reporter Target of test results.
|
|
* @return SimpleTestXmlListener XML reader.
|
|
* @access protected
|
|
*/
|
|
function &_createParser(&$reporter) {
|
|
return new SimpleTestXmlParser($reporter);
|
|
}
|
|
|
|
/**
|
|
* Accessor for the number of subtests.
|
|
* @return integer Number of test cases.
|
|
* @access public
|
|
*/
|
|
function getSize() {
|
|
if ($this->_size === false) {
|
|
$browser = &$this->_createBrowser();
|
|
$xml = $browser->get($this->_dry_url);
|
|
if (! $xml) {
|
|
trigger_error('Cannot read remote test URL [' . $this->_dry_url . ']');
|
|
return false;
|
|
}
|
|
$reporter = &new SimpleReporter();
|
|
$parser = &$this->_createParser($reporter);
|
|
if (! $parser->parse($xml)) {
|
|
trigger_error('Cannot parse incoming XML from [' . $this->_dry_url . ']');
|
|
return false;
|
|
}
|
|
$this->_size = $reporter->getTestCaseCount();
|
|
}
|
|
return $this->_size;
|
|
}
|
|
}
|
|
?>
|