mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2024-11-15 11:28:25 +00:00
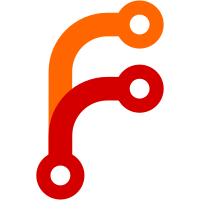
Changing version number to 1.x.x.x, 0.10.x.x code base had been changed to the version number 1.x.x.x, and what was planned for 1.x.x.x code has now been moved to 2.x.x.x, and 2.x.x.x moved to 3.x.x.x. This will give us easier to track version numbers from now on. Revision: [2248] Merging changes from model_php5.php Revision: [2247] "Removing test code from view class" Revision: [2246] Removed cache time define from core.php. Modified the __() function in basics.php to echo string like it will in later versions of cake with translations. Refactored the cache checking in bootstrap.php to read the files embedded time stamp and delete or output the cached version. Added View::cacheView() for caching pages. Revision: [2245] Moving column formatting from DBO to Sanitize Revision: [2244] Adding beforeValidate() Model callback, and allowing query data to be modified in beforeFind() Revision: [2243] "Adding caching changes to Controller class " Revision: [2242] "Added check to delete cached version if it has expired" Revision: [2241] Adding app/cache/views directory Revision: [2240] "Fixed missing variable" Revision: [2239] "Adding full page caching to view class." Revision: [2238] "Adding defines for caching" Revision: [2237] "Adding caching check too bootstrap.php" Revision: [2236] Adding ClassRegistry::removeObject from Ticket #477 Revision: [2235] "Correcting setting in DATABASE_CONFIG class" Revision: [2231] Adding convenience function am(), which allows merging an infinite number of arrays merged into one Revision: [2207] Change Model::save() to call beforeSave() before validations Revision: [2199] Removing conditions method call in Model::field() Revision: [2196] Setting proper mime type again git-svn-id: https://svn.cakephp.org/repo/trunk/cake@2250 3807eeeb-6ff5-0310-8944-8be069107fe0
128 lines
No EOL
2.9 KiB
PHP
128 lines
No EOL
2.9 KiB
PHP
<?php
|
|
/* SVN FILE: $Id$ */
|
|
|
|
/**
|
|
* Class collections.
|
|
*
|
|
* A repository for class objects, each registered with a key.
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright (c) 2006, Cake Software Foundation, Inc.
|
|
* 1785 E. Sahara Avenue, Suite 490-204
|
|
* Las Vegas, Nevada 89104
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @copyright Copyright (c) 2006, Cake Software Foundation, Inc.
|
|
* @link http://www.cakefoundation.org/projects/info/cakephp CakePHP Project
|
|
* @package cake
|
|
* @subpackage cake.cake.libs
|
|
* @since CakePHP v 0.9.2
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
|
|
/**
|
|
* Class Collections.
|
|
*
|
|
* A repository for class objects, each registered with a key.
|
|
* If you try to add an object with the same key twice, nothing will come of it.
|
|
* If you need a second instance of an object, give it another key.
|
|
*
|
|
* @package cake
|
|
* @subpackage cake.cake.libs
|
|
* @since CakePHP v 0.9.2
|
|
*/
|
|
class ClassRegistry
|
|
{
|
|
|
|
/**
|
|
* Names of classes with their objects.
|
|
*
|
|
* @var array
|
|
* @access private
|
|
*/
|
|
var $_objects = array();
|
|
|
|
/**
|
|
* Return a singleton instance of the ClassRegistry.
|
|
*
|
|
* @return ClassRegistry instance
|
|
*/
|
|
function &getInstance()
|
|
{
|
|
static $instance = array();
|
|
if (!$instance)
|
|
{
|
|
$instance[0] =& new ClassRegistry;
|
|
}
|
|
return $instance[0];
|
|
}
|
|
|
|
/**
|
|
* Add $object to the registry, associating it with the name $key.
|
|
*
|
|
* @param string $key
|
|
* @param mixed $object
|
|
*/
|
|
function addObject($key, &$object)
|
|
{
|
|
$_this =& ClassRegistry::getInstance();
|
|
$key = low($key);
|
|
|
|
if (array_key_exists($key, $_this->_objects) === false)
|
|
{
|
|
$_this->_objects[$key] =& $object;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Remove object which corresponds to given key.
|
|
*
|
|
* @param string $key
|
|
* @return void
|
|
*/
|
|
function removeObject($key)
|
|
{
|
|
$_this =& ClassRegistry::getInstance();
|
|
$key = low($key);
|
|
|
|
if (array_key_exists($key, $_this->_objects) === true)
|
|
{
|
|
unset($_this->_objects[$key]);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Returns true if given key is present in the ClassRegistry.
|
|
*
|
|
* @param string $key Key to look for
|
|
* @return boolean Success
|
|
*/
|
|
function isKeySet($key)
|
|
{
|
|
$_this =& ClassRegistry::getInstance();
|
|
$key = strtolower($key);
|
|
return array_key_exists($key, $_this->_objects);
|
|
}
|
|
|
|
/**
|
|
* Return object which corresponds to given key.
|
|
*
|
|
* @param string $key
|
|
* @return mixed
|
|
*/
|
|
function &getObject($key)
|
|
{
|
|
$key = strtolower($key);
|
|
$_this =& ClassRegistry::getInstance();
|
|
return $_this->_objects[$key];
|
|
}
|
|
}
|
|
?>
|