mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2025-01-31 09:06:17 +00:00
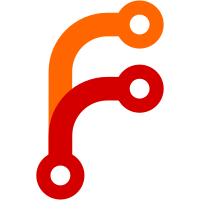
helpers can now use this like a url to get methods return value: $helpername->renderMethod('/controller/action/param/'); Refactored dispatcher. Adding bug fix by nate in [894] [896] Added extra param View::renderMethod() Fixed bug related to #72, #111, #113, #209 Some of those tickets where closed as duplicates already git-svn-id: https://svn.cakephp.org/repo/trunk/cake@900 3807eeeb-6ff5-0310-8944-8be069107fe0
193 lines
No EOL
5.1 KiB
PHP
193 lines
No EOL
5.1 KiB
PHP
<?php
|
|
/* SVN FILE: $Id$ */
|
|
|
|
/**
|
|
* Short description for file.
|
|
*
|
|
* Long description for file
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright (c) 2005, CakePHP Authors/Developers
|
|
*
|
|
* Author(s): Michal Tatarynowicz aka Pies <tatarynowicz@gmail.com>
|
|
* Larry E. Masters aka PhpNut <nut@phpnut.com>
|
|
* Kamil Dzielinski aka Brego <brego.dk@gmail.com>
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @author CakePHP Authors/Developers
|
|
* @copyright Copyright (c) 2005, CakePHP Authors/Developers
|
|
* @link https://trac.cakephp.org/wiki/Authors Authors/Developers
|
|
* @package cake
|
|
* @subpackage cake.libs
|
|
* @since CakePHP v 0.2.9
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
|
|
/**
|
|
* Backend for helpers.
|
|
*
|
|
* Long description for class
|
|
*
|
|
* @package cake
|
|
* @subpackage cake.libs
|
|
* @since CakePHP v 0.9.2
|
|
*
|
|
*/
|
|
class Helper extends Object
|
|
{
|
|
/*************************************************************************
|
|
* Public variables
|
|
*************************************************************************/
|
|
|
|
/**#@+
|
|
* @access public
|
|
*/
|
|
|
|
|
|
/**
|
|
* Holds tag templates.
|
|
*
|
|
* @access public
|
|
* @var array
|
|
*/
|
|
var $tags = array();
|
|
|
|
/**#@-*/
|
|
|
|
/*************************************************************************
|
|
* Public methods
|
|
*************************************************************************/
|
|
|
|
/**#@+
|
|
* @access public
|
|
*/
|
|
|
|
/**
|
|
* Constructor.
|
|
*
|
|
* Parses tag templates into $this->tags.
|
|
*
|
|
* @return void
|
|
*/
|
|
function Helper()
|
|
{
|
|
$this->tags = $this->readConfigFile(CONFIGS.'tags.ini.php');
|
|
}
|
|
|
|
/**
|
|
* Decides wheter to output or return a string.
|
|
*
|
|
* Based on AUTO_OUTPUT and $return's value, this method decides wheter to
|
|
* output a string, or return it.
|
|
*
|
|
* @param string $str String to be outputed or returned.
|
|
* @param boolean $return Wheter this method should return a value or
|
|
* output it. This overrides AUTO_OUTPUT.
|
|
* @return mixed Either string or boolean value, depends on AUTO_OUTPUT
|
|
* and $return.
|
|
*/
|
|
function output($str, $return = false)
|
|
{
|
|
if (AUTO_OUTPUT && $return === false)
|
|
{
|
|
if (print $str)
|
|
{
|
|
return true;
|
|
}
|
|
else
|
|
{
|
|
return false;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
return $str;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Assigns values to tag templates.
|
|
*
|
|
* Finds a tag template by $keyName, and replaces $values's keys with
|
|
* $values's keys.
|
|
*
|
|
* @param string $keyName Name of the key in the tag array.
|
|
* @param array $values Values to be inserted into tag.
|
|
* @return string Tag with inserted values.
|
|
*/
|
|
function assign($keyName, $values)
|
|
{
|
|
return str_replace('%%'.array_keys($values).'%%', array_values($values),
|
|
$this->tags[$keyName]);
|
|
}
|
|
|
|
|
|
/**
|
|
*
|
|
* Allow calling a controllers method from a helper
|
|
*
|
|
*
|
|
* @param unknown_type $url
|
|
* @param unknown_type $extra
|
|
* @return unknown
|
|
*/
|
|
function renderMethod ($url, $extra = false)
|
|
{
|
|
$dispatcher = new Dispatcher();
|
|
return $dispatcher->dispatch($url, array('bare'=>1));
|
|
}
|
|
|
|
function readConfigFile ($fileName)
|
|
{
|
|
$fileLineArray = file($fileName);
|
|
|
|
foreach ($fileLineArray as $fileLine)
|
|
{
|
|
$dataLine = trim($fileLine);
|
|
$firstChar = substr($dataLine, 0, 1);
|
|
if ($firstChar != ';' && $dataLine != '')
|
|
{
|
|
if ($firstChar == '[' && substr($dataLine, -1, 1) == ']')
|
|
{
|
|
// [section block] we might use this later do not know for sure
|
|
// this could be used to add a key with the section block name
|
|
// but it adds another array level
|
|
}
|
|
else
|
|
{
|
|
$delimiter = strpos($dataLine, '=');
|
|
if ($delimiter > 0)
|
|
{
|
|
$key = strtolower(trim(substr($dataLine, 0, $delimiter)));
|
|
$value = trim(substr($dataLine, $delimiter + 1));
|
|
if (substr($value, 0, 1) == '"' && substr($value, -1) == '"')
|
|
{
|
|
$value = substr($value, 1, -1);
|
|
}
|
|
$iniSetting[$key] = stripcslashes($value);
|
|
}
|
|
else
|
|
{
|
|
$iniSetting[strtolower(trim($dataLine))]='';
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
}
|
|
}
|
|
return $iniSetting;
|
|
}
|
|
|
|
/**#@-*/
|
|
}
|
|
|
|
?>
|