mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2025-01-20 03:26:16 +00:00
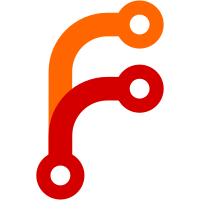
The option to sort has been removed and the list of shells is now sorted and grouped by plugin and then by command. Core and app shells are always listed last.
118 lines
2.8 KiB
PHP
118 lines
2.8 KiB
PHP
<?php
|
|
/**
|
|
* CommandListShellTest file
|
|
*
|
|
* PHP 5
|
|
*
|
|
* CakePHP : Rapid Development Framework (http://cakephp.org)
|
|
* Copyright 2005-2012, Cake Software Foundation, Inc.
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @copyright Copyright 2005-2012, Cake Software Foundation, Inc.
|
|
* @link http://cakephp.org CakePHP Project
|
|
* @package Cake.Test.Case.Console.Command
|
|
* @since CakePHP v 2.0
|
|
* @license MIT License (http://www.opensource.org/licenses/mit-license.php)
|
|
*/
|
|
|
|
App::uses('CommandListShell', 'Console/Command');
|
|
App::uses('ConsoleOutput', 'Console');
|
|
App::uses('ConsoleInput', 'Console');
|
|
App::uses('Shell', 'Console');
|
|
|
|
|
|
class TestStringOutput extends ConsoleOutput {
|
|
|
|
public $output = '';
|
|
|
|
protected function _write($message) {
|
|
$this->output .= $message;
|
|
}
|
|
|
|
}
|
|
|
|
class CommandListShellTest extends CakeTestCase {
|
|
|
|
/**
|
|
* setUp method
|
|
*
|
|
* @return void
|
|
*/
|
|
public function setUp() {
|
|
parent::setUp();
|
|
App::build(array(
|
|
'Plugin' => array(
|
|
CAKE . 'Test' . DS . 'test_app' . DS . 'Plugin' . DS
|
|
),
|
|
'Console/Command' => array(
|
|
CAKE . 'Test' . DS . 'test_app' . DS . 'Console' . DS . 'Command' . DS
|
|
)
|
|
), App::RESET);
|
|
CakePlugin::load(array('TestPlugin', 'TestPluginTwo'));
|
|
|
|
$out = new TestStringOutput();
|
|
$in = $this->getMock('ConsoleInput', array(), array(), '', false);
|
|
|
|
$this->Shell = $this->getMock(
|
|
'CommandListShell',
|
|
array('in', '_stop', 'clear'),
|
|
array($out, $out, $in)
|
|
);
|
|
}
|
|
|
|
/**
|
|
* tearDown
|
|
*
|
|
* @return void
|
|
*/
|
|
public function tearDown() {
|
|
parent::tearDown();
|
|
unset($this->Shell);
|
|
CakePlugin::unload();
|
|
}
|
|
|
|
/**
|
|
* test that main finds core shells.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function testMain() {
|
|
$this->Shell->main();
|
|
$output = $this->Shell->stdout->output;
|
|
|
|
$expected = "/\[.*TestPlugin.*\] example/";
|
|
$this->assertRegExp($expected, $output);
|
|
|
|
$expected = "/\[.*TestPluginTwo.*\] example, welcome/";
|
|
$this->assertRegExp($expected, $output);
|
|
|
|
$expected = "/\[.*CORE.*\] acl, api, bake, command_list, console, i18n, schema, test, testsuite, upgrade/";
|
|
$this->assertRegExp($expected, $output);
|
|
|
|
$expected = "/\[.*app.*\] sample/";
|
|
$this->assertRegExp($expected, $output);
|
|
}
|
|
|
|
/**
|
|
* test xml output.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function testMainXml() {
|
|
$this->Shell->params['xml'] = true;
|
|
$this->Shell->main();
|
|
|
|
$output = $this->Shell->stdout->output;
|
|
|
|
$find = '<shell name="sample" call_as="sample" provider="app" help="sample -h"/>';
|
|
$this->assertContains($find, $output);
|
|
|
|
$find = '<shell name="bake" call_as="bake" provider="CORE" help="bake -h"/>';
|
|
$this->assertContains($find, $output);
|
|
|
|
$find = '<shell name="welcome" call_as="TestPluginTwo.welcome" provider="TestPluginTwo" help="TestPluginTwo.welcome -h"/>';
|
|
$this->assertContains($find, $output);
|
|
}
|
|
}
|