mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2024-11-15 19:38:26 +00:00
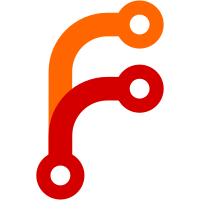
Revision: [1936] Adding fix for Ticket #364 Revision: [1935] Adding script.ac slider control and fixing Ticket #188 for real this time Revision: [1934] [1933] [1932] [1931] [1930] Docstrings, and some inconsistency fixed. Wrong parameters in one case. Moved them into the signature. Also some code standards fiddling. Some outdated parameters. Added a todo in docstrings. git-svn-id: https://svn.cakephp.org/repo/trunk/cake@1937 3807eeeb-6ff5-0310-8944-8be069107fe0
166 lines
No EOL
3.2 KiB
PHP
166 lines
No EOL
3.2 KiB
PHP
<?php
|
|
/* SVN FILE: $Id$ */
|
|
|
|
/**
|
|
* Access Control List factory class.
|
|
*
|
|
* Permissions system.
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright (c) 2006, Cake Software Foundation, Inc.
|
|
* 1785 E. Sahara Avenue, Suite 490-204
|
|
* Las Vegas, Nevada 89104
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @copyright Copyright (c) 2006, Cake Software Foundation, Inc.
|
|
* @link http://www.cakefoundation.org/projects/info/cakephp CakePHP Project
|
|
* @package cake
|
|
* @subpackage cake.cake.libs.controller.components
|
|
* @since CakePHP v 0.10.0.1076
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
|
|
/**
|
|
* Access Control List factory class.
|
|
*
|
|
* Looks for ACL implementation class in core config, and returns an instance of that class.
|
|
*
|
|
* @package cake
|
|
* @subpackage cake.cake.libs.controller.components
|
|
* @since CakePHP v 0.10.0.1076
|
|
*
|
|
*/
|
|
class AclComponent extends Object
|
|
{
|
|
|
|
var $_instance = null;
|
|
var $controller = true;
|
|
|
|
/**
|
|
* Constructor. Will return an instance of the correct ACL class.
|
|
*
|
|
*/
|
|
function __construct()
|
|
{
|
|
$this->getACL();
|
|
}
|
|
|
|
/**
|
|
* Static function used to gain an instance of the correct ACL class.
|
|
*
|
|
* @return MyACL
|
|
*/
|
|
function &getACL()
|
|
{
|
|
if($this->_instance == null)
|
|
{
|
|
uses('controller'.DS.'components'.DS.ACL_FILENAME);
|
|
$classname = ACL_CLASSNAME;
|
|
$this->_instance = new $classname;
|
|
}
|
|
return $this->_instance;
|
|
}
|
|
|
|
/**
|
|
* Empty class defintion, to be overridden in subclasses.
|
|
*
|
|
*/
|
|
function _initACL()
|
|
{
|
|
|
|
}
|
|
|
|
/**
|
|
* Pass-thru function for ACL check instance.
|
|
*
|
|
* @return boolean
|
|
*/
|
|
function check($aro, $aco, $action = "*")
|
|
{
|
|
return $this->_instance->check($aro, $aco, $action);
|
|
}
|
|
|
|
|
|
/**
|
|
* Pass-thru function for ACL allow instance.
|
|
*
|
|
* @return boolean
|
|
*/
|
|
function allow($aro, $aco, $action = "*")
|
|
{
|
|
return $this->_instance->allow($aro, $aco, $action);
|
|
}
|
|
|
|
/**
|
|
* Pass-thru function for ACL deny instance.
|
|
*
|
|
* @return boolean
|
|
*/
|
|
function deny($aro, $aco, $action = "*")
|
|
{
|
|
return $this->_instance->deny($aro, $aco, $action);
|
|
}
|
|
|
|
/**
|
|
* Pass-thru function for ACL inherit instance.
|
|
*
|
|
* @return boolean
|
|
*/
|
|
function inherit($aro, $aco, $action = "*")
|
|
{
|
|
return $this->_instance->inherit($aro, $aco, $action);
|
|
}
|
|
|
|
/**
|
|
* Pass-thru function for ACL grant instance.
|
|
*
|
|
* @return boolean
|
|
*/
|
|
function grant($aro, $aco, $action = "*")
|
|
{
|
|
return $this->_instance->grant($aro, $aco, $action);
|
|
}
|
|
|
|
/**
|
|
* Pass-thru function for ACL grant instance.
|
|
*
|
|
* @return boolean
|
|
*/
|
|
function revoke($aro, $aco, $action = "*")
|
|
{
|
|
return $this->_instance->revoke($aro, $aco, $action);
|
|
}
|
|
|
|
|
|
/**
|
|
* Pass-thru function for ACL getAro instance.
|
|
*
|
|
* @return Aro
|
|
*/
|
|
function getAro($id)
|
|
{
|
|
return $this->_instance->getAro($id);
|
|
}
|
|
|
|
|
|
/**
|
|
* Pass-thru function for ACL getAco instance.
|
|
*
|
|
* @return Aco
|
|
*/
|
|
function getAco($id)
|
|
{
|
|
return $this->_instance->getAco($id);
|
|
}
|
|
|
|
}
|
|
|
|
?>
|