mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2025-01-19 11:06:15 +00:00
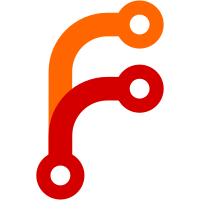
Revision: [1985] Changed DboSource?::order() to allow passing an array in the order param Revision: [1984] Reverting changes from [1983] Revision: [1983] Merging change from [1966] and [1967] Revision: [1982] Adding fix for DboSource::order(). This allows setting the order in the find methods. Revision: [1981] cleaned up code Revision: [1980] Corrected the array keys in the regex I added Revision: [1979] Added check to DboMysql::value() that does not add quotes around a numerical value. Refactored DboSource::conditions() adding better regex. Revision: [1978] Added check for LIKE in a condition array this fixes the = being added. Revision: [1977] Added fix for Ticket #392 Revision: [1976] Adding changes suggested in Ticket #381. These have not been fully tested. Revision: [1975] Added fix for Ticket #391 Revision: [1974] Added patch from Ticket #390 Revision: [1973] Adding patch from Ticket #386 Revision: [1972] Added patch from Ticket #385. Changed wording of a comment. Revision: [1971] Added patch from Ticket #383 Revision: [1970] Adding fix for Ticket #395 Revision: [1969] Adding more detailed comment to path defines Revision: [1968] Making a few more changes to the path settings Revision: [1965] fixing path issue with loading PagesController Revision: [1964] Added model method for getting column types by field Revision: [1963] Corrected paths to the tmp directory. Making a few more changes to the defines in index.php Revision: [1962] Moving tmp directory to app Revision: [1961] Starting separation of core from the application. Revision: [1960] Adding vendors directory to app directory Revision: [1959] Finished support for recursive associations. Still needs some testing... Revision: [1958] Adding fix for Ticket #387, and automagic id's for form inputs Revision: [1957] Revision: [1956] Adding fix for error reported in Google Group: http://groups.google.com/group/cake-php/browse_thread/thread/395593a3cea34174 Revision: [1955] Adding fix for Controller::referer() git-svn-id: https://svn.cakephp.org/repo/trunk/cake@1986 3807eeeb-6ff5-0310-8944-8be069107fe0
184 lines
No EOL
4.7 KiB
PHP
184 lines
No EOL
4.7 KiB
PHP
<?php
|
|
/* SVN FILE: $Id$ */
|
|
|
|
/**
|
|
* Backend for helpers.
|
|
*
|
|
* Internal methods for the Helpers.
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright (c) 2006, Cake Software Foundation, Inc.
|
|
* 1785 E. Sahara Avenue, Suite 490-204
|
|
* Las Vegas, Nevada 89104
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @copyright Copyright (c) 2006, Cake Software Foundation, Inc.
|
|
* @link http://www.cakefoundation.org/projects/info/cakephp CakePHP Project
|
|
* @package cake
|
|
* @subpackage cake.cake.libs.view
|
|
* @since CakePHP v 0.2.9
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
|
|
/**
|
|
* Backend for helpers.
|
|
*
|
|
* Long description for class
|
|
*
|
|
* @package cake
|
|
* @subpackage cake.cake.libs.view
|
|
* @since CakePHP v 0.9.2
|
|
*
|
|
*/
|
|
class Helper extends Object
|
|
{
|
|
/*************************************************************************
|
|
* Public variables
|
|
*************************************************************************/
|
|
|
|
/**#@+
|
|
* @access public
|
|
*/
|
|
|
|
|
|
/**
|
|
* Holds tag templates.
|
|
*
|
|
* @access public
|
|
* @var array
|
|
*/
|
|
var $tags = array();
|
|
|
|
/**#@-*/
|
|
|
|
/*************************************************************************
|
|
* Public methods
|
|
*************************************************************************/
|
|
|
|
/**#@+
|
|
* @access public
|
|
*/
|
|
|
|
/**
|
|
* Constructor.
|
|
*
|
|
* Parses tag templates into $this->tags.
|
|
*
|
|
* @return void
|
|
*/
|
|
function Helper()
|
|
{
|
|
}
|
|
|
|
function loadConfig()
|
|
{
|
|
return $this->readConfigFile($config = fileExistsInPath(CAKE.'config'.DS.'tags.ini.php'));
|
|
}
|
|
|
|
/**
|
|
* Decides whether to output or return a string.
|
|
*
|
|
* Based on AUTO_OUTPUT and $return's value, this method decides whether to
|
|
* output a string, or return it.
|
|
*
|
|
* @param string $str String to be output or returned.
|
|
* @param boolean $return Whether this method should return a value or
|
|
* output it. This overrides AUTO_OUTPUT.
|
|
* @return mixed Either string or boolean value, depends on AUTO_OUTPUT
|
|
* and $return.
|
|
*/
|
|
function output($str, $return = false)
|
|
{
|
|
if (AUTO_OUTPUT && $return === false)
|
|
{
|
|
if (print $str)
|
|
{
|
|
return true;
|
|
}
|
|
else
|
|
{
|
|
return false;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
return $str;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Assigns values to tag templates.
|
|
*
|
|
* Finds a tag template by $keyName, and replaces $values's keys with
|
|
* $values's keys.
|
|
*
|
|
* @param string $keyName Name of the key in the tag array.
|
|
* @param array $values Values to be inserted into tag.
|
|
* @return string Tag with inserted values.
|
|
*/
|
|
function assign($keyName, $values)
|
|
{
|
|
return str_replace('%%'.array_keys($values).'%%', array_values($values),
|
|
$this->tags[$keyName]);
|
|
}
|
|
|
|
/**
|
|
* Returns an array of settings in given INI file.
|
|
*
|
|
* @param string $fileName
|
|
* @return array
|
|
*/
|
|
function readConfigFile ($fileName)
|
|
{
|
|
$fileLineArray = file($fileName);
|
|
|
|
foreach ($fileLineArray as $fileLine)
|
|
{
|
|
$dataLine = trim($fileLine);
|
|
$firstChar = substr($dataLine, 0, 1);
|
|
if ($firstChar != ';' && $dataLine != '')
|
|
{
|
|
if ($firstChar == '[' && substr($dataLine, -1, 1) == ']')
|
|
{
|
|
// [section block] we might use this later do not know for sure
|
|
// this could be used to add a key with the section block name
|
|
// but it adds another array level
|
|
}
|
|
else
|
|
{
|
|
$delimiter = strpos($dataLine, '=');
|
|
if ($delimiter > 0)
|
|
{
|
|
$key = strtolower(trim(substr($dataLine, 0, $delimiter)));
|
|
$value = trim(substr($dataLine, $delimiter + 1));
|
|
if (substr($value, 0, 1) == '"' && substr($value, -1) == '"')
|
|
{
|
|
$value = substr($value, 1, -1);
|
|
}
|
|
$iniSetting[$key] = stripcslashes($value);
|
|
}
|
|
else
|
|
{
|
|
$iniSetting[strtolower(trim($dataLine))]='';
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
}
|
|
}
|
|
return $iniSetting;
|
|
}
|
|
|
|
/**#@-*/
|
|
}
|
|
|
|
?>
|