mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2025-03-19 16:10:54 +00:00
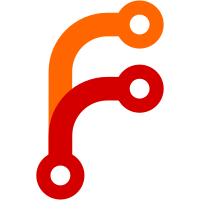
Added ability to turn off HTTP_USER_AGENT check in a Controller::beforeFilter(), Added id() to Session helper and component to return current Session id, the component accepts a $id parameter to force setting the Session id which must be called in a Controller::beforeFilter(). Sessions id are not longer renewed if a request is from Ajax, or from requestAction(); When Security.level (1.2) or CAKE_SECURITY (1.1) is set the 'high' renewing of Session id only happens if request is 2 seconds after the last request. Added $_Session[Config][timeout] which forces renewing Session if request are within the 2 second limit and over 10 request. If an application is expected to make multiple request (more than 10) to the server in a single proccess, Configure::write('Security.level', 'medium'); (1.2) or $this->Session->security = 'medium'; (1.1) should be used in a beforeFilter for the specific methods. 1.2 Sessions allow using CacheEngines to store Sessions, be aware that using memory caching as the only storage of Sessions is not reliable. Further work will be done to allow using the CacheEngines with database Sessions, etc. git-svn-id: https://svn.cakephp.org/repo/branches/1.2.x.x@5982 3807eeeb-6ff5-0310-8944-8be069107fe0
140 lines
No EOL
4.1 KiB
PHP
140 lines
No EOL
4.1 KiB
PHP
<?php
|
|
/* SVN FILE: $Id$ */
|
|
/**
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP(tm) : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright 2005-2007, Cake Software Foundation, Inc.
|
|
* 1785 E. Sahara Avenue, Suite 490-204
|
|
* Las Vegas, Nevada 89104
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @copyright Copyright 2005-2007, Cake Software Foundation, Inc.
|
|
* @link http://www.cakefoundation.org/projects/info/cakephp CakePHP(tm) Project
|
|
* @package cake
|
|
* @subpackage cake.cake.libs.controller
|
|
* @since CakePHP(tm) v TBD
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
/**
|
|
* Base class for all CakePHP Components.
|
|
*
|
|
* @package cake
|
|
* @subpackage cake.cake.libs.controller
|
|
*/
|
|
class Component extends Object {
|
|
/**
|
|
* Components used by this component.
|
|
*
|
|
* @var array
|
|
* @access public
|
|
*/
|
|
var $components = array();
|
|
/**
|
|
* Controller to which this component is linked.
|
|
*
|
|
* @var object
|
|
* @access public
|
|
*/
|
|
var $controller = null;
|
|
|
|
/**
|
|
* Constructor
|
|
*
|
|
* @return object
|
|
*/
|
|
function __construct() {
|
|
}
|
|
/**
|
|
* Used to initialize the components for current controller
|
|
*
|
|
* @param object $controller Controller using this component.
|
|
* @access public
|
|
*/
|
|
function init(&$controller) {
|
|
$this->controller =& $controller;
|
|
if ($this->controller->components !== false) {
|
|
$loaded = array();
|
|
$this->controller->components = array_merge(array('Session'), $this->controller->components);
|
|
$loaded = $this->_loadComponents($loaded, $this->controller->components);
|
|
|
|
foreach (array_keys($loaded) as $component) {
|
|
$tempComponent =& $loaded[$component];
|
|
if (isset($tempComponent->components) && is_array($tempComponent->components)) {
|
|
foreach ($tempComponent->components as $subComponent) {
|
|
$this->controller->{$component}->{$subComponent} =& $loaded[$subComponent];
|
|
}
|
|
}
|
|
if (is_callable(array($tempComponent, 'initialize'))) {
|
|
$tempComponent->initialize($controller);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
/**
|
|
* Load components used by this component.
|
|
*
|
|
* @param array $loaded Components already loaded (indexed by component name)
|
|
* @param array $components Components to load
|
|
* @return array Components loaded
|
|
* @access protected
|
|
*/
|
|
function &_loadComponents(&$loaded, $components) {
|
|
foreach ($components as $component) {
|
|
$parts = preg_split('/\/|\./', $component);
|
|
|
|
if (count($parts) === 1) {
|
|
$plugin = $this->controller->plugin;
|
|
} else {
|
|
$plugin = Inflector::underscore($parts['0']);
|
|
$component = $parts[count($parts) - 1];
|
|
}
|
|
|
|
$componentCn = $component . 'Component';
|
|
|
|
if (in_array($component, array_keys($loaded)) !== true) {
|
|
if (!class_exists($componentCn)) {
|
|
if (is_null($plugin) || !loadPluginComponent($plugin, $component)) {
|
|
if (!loadComponent($component)) {
|
|
$this->cakeError('missingComponentFile', array(array(
|
|
'className' => $this->controller->name,
|
|
'component' => $component,
|
|
'file' => Inflector::underscore($component) . '.php',
|
|
'base' => $this->controller->base)));
|
|
exit();
|
|
}
|
|
}
|
|
|
|
if (!class_exists($componentCn)) {
|
|
$this->cakeError('missingComponentClass', array(array(
|
|
'className' => $this->controller->name,
|
|
'component' => $component,
|
|
'file' => Inflector::underscore($component) . '.php',
|
|
'base' => $this->controller->base)));
|
|
exit();
|
|
}
|
|
}
|
|
|
|
if ($componentCn == 'SessionComponent') {
|
|
$param = Router::stripPlugin($this->controller->base, $this->controller->plugin) . '/';
|
|
} else {
|
|
$param = null;
|
|
}
|
|
$this->controller->{$component} =& new $componentCn($param);
|
|
$loaded[$component] =& $this->controller->{$component};
|
|
if (isset($this->controller->{$component}->components) && is_array($this->controller->{$component}->components)) {
|
|
$loaded =& $this->_loadComponents($loaded, $this->controller->{$component}->components);
|
|
}
|
|
}
|
|
}
|
|
return $loaded;
|
|
}
|
|
}
|
|
?>
|