mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2025-02-07 12:36:25 +00:00
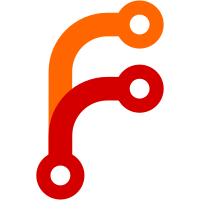
Adding empty directories where overrides for the core views can be placed. Adding an empty directory for elements [1127] Adding directory to hold core inflection files [1128] More work on the new inflector. This still is not completed but should be soon [1130] Documentation strings, du jour. [1131] Docstringed and ready. Inflector lacks one docstring. It is noted in its todo [1132] Incomplete documentation, and some corrections to previous documentation. Gwoo noted that there'll be more changes in the Helpers soon, so I back off here. [1134] Adding before filters back to code. Commented out a regex in Inflector::pluralize(); that os causing problems. Removed a duplicate define in index.php. Removed the bare array being set automatically when using requestAction(). With this change you must use requestAction(); like this. $object->requestAction('/bare/controller/action/param'); Added GOTCHAS file with links to problems people may have with CakePHP. Some more work done on new Inflector. [1135] Added a check when trying to access a private method of a controller. This will now display an error page informing user that this is not allowed. [1137] Fixed a few undefined variable errors in the code Corrected problem with double layout display when an error is returned and caught. git-svn-id: https://svn.cakephp.org/repo/trunk/cake@1138 3807eeeb-6ff5-0310-8944-8be069107fe0
244 lines
No EOL
5.6 KiB
PHP
244 lines
No EOL
5.6 KiB
PHP
<?php
|
|
/* SVN FILE: $Id$ */
|
|
|
|
/**
|
|
* Short description for file.
|
|
*
|
|
* Long description for file
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright (c) 2005, CakePHP Authors/Developers
|
|
*
|
|
* Author(s): Michal Tatarynowicz aka Pies <tatarynowicz@gmail.com>
|
|
* Larry E. Masters aka PhpNut <nut@phpnut.com>
|
|
* Kamil Dzielinski aka Brego <brego.dk@gmail.com>
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @author CakePHP Authors/Developers
|
|
* @copyright Copyright (c) 2005, CakePHP Authors/Developers
|
|
* @link https://trac.cakephp.org/wiki/Authors Authors/Developers
|
|
* @package cake
|
|
* @subpackage cake.cake.libs
|
|
* @since CakePHP v .0.10.x.x
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
|
|
/**
|
|
* Short description for class
|
|
*
|
|
* Inflector pluralizes and singularizes English words.
|
|
*
|
|
* @package cake
|
|
* @subpackage cake.cake.libs
|
|
* @since CakePHP v .0.10.x.x
|
|
*/
|
|
class Inflector
|
|
{
|
|
var $classical = array();
|
|
|
|
var $pre = '';
|
|
|
|
var $word = '';
|
|
|
|
var $post = '';
|
|
|
|
|
|
function &getInstance() {
|
|
|
|
static $instance = array();
|
|
if (!$instance)
|
|
{
|
|
$instance[0] =& new Inflector;
|
|
}
|
|
return $instance[0];
|
|
}
|
|
|
|
function pluralize($text, $type = 'Noun' , $classical = false)
|
|
{
|
|
$inflec =& Inflector::getInstance();
|
|
$inflec->classical = $classical;
|
|
$inflec->count = strlen($text);
|
|
|
|
if ($inflec->count == 1)
|
|
{
|
|
return $text;
|
|
}
|
|
if(empty($text))
|
|
{
|
|
return;
|
|
}
|
|
|
|
$inflec->_pre($text);
|
|
|
|
if (empty($inflec->word))
|
|
{
|
|
return $text;
|
|
}
|
|
|
|
$type = '_plural'.$type;
|
|
$inflected = $inflec->_postProcess($inflec->word,$inflec->$type());
|
|
return $inflected;
|
|
}
|
|
|
|
function singularize($text, $type = 'Noun' , $classical = false)
|
|
{
|
|
$inflec =& Inflector::getInstance();
|
|
$inflec->classical = $classical;
|
|
$inflec->count = count($text);
|
|
|
|
if ($inflec->count == 1)
|
|
{
|
|
return $text;
|
|
}
|
|
if(empty($text))
|
|
{
|
|
return;
|
|
}
|
|
|
|
return $inflec->_singular.$type($text);
|
|
}
|
|
|
|
function _pluralNoun()
|
|
{
|
|
$inflec =& Inflector::getInstance();
|
|
|
|
require_once('config/nouns.php');
|
|
|
|
$regexPluralUninflected = $inflec->_enclose(join( '|', array_values(array_merge($pluralUninflected,$pluralUninflecteds))));
|
|
|
|
$regexPluralUninflectedHerd = $inflec->_enclose(join( '|', array_values($pluralUninflectedHerd)));
|
|
|
|
$pluralIrregular = array_merge($pluralIrregular,$pluralIrregulars);
|
|
$regexPluralIrregular = $inflec->_enclose(join( '|', array_keys($pluralIrregular)));
|
|
|
|
if (preg_match('/^('.$regexPluralUninflected.')$/i', $inflec->word, $regs))
|
|
{
|
|
return $inflec->word;
|
|
}
|
|
|
|
if (empty($inflec->classical))
|
|
{
|
|
preg_match('/^('.$regexPluralUninflectedHerd.')$/i', $inflec->word, $regs);
|
|
return $inflec->word;
|
|
}
|
|
|
|
if (preg_match('/(.*)\\b('.$regexPluralIrregular.')$/i', $inflec->word, $regs))
|
|
{
|
|
return $regs[1] . $pluralIrregular[strtolower($regs[2])];
|
|
}
|
|
|
|
return $inflec->word.'s';
|
|
}
|
|
|
|
function _pluralVerb($text)
|
|
{
|
|
return $pluralText;
|
|
}
|
|
|
|
function _pluralAdjective($text)
|
|
{
|
|
return $pluralText;
|
|
}
|
|
|
|
function _pluralSpecialNoun($text)
|
|
{
|
|
return $pluralText;
|
|
}
|
|
|
|
function _pluralSpecialVerb($text)
|
|
{
|
|
return $pluralText;
|
|
}
|
|
|
|
function _pluralGeneralVerb($text)
|
|
{
|
|
return $pluralText;
|
|
}
|
|
|
|
function _pluralSpecialAdjective($text)
|
|
{
|
|
return $pluralText;
|
|
}
|
|
|
|
function _singularNoun($text)
|
|
{
|
|
return $text;
|
|
}
|
|
|
|
function _singularVerb($text)
|
|
{
|
|
return $singularText;
|
|
}
|
|
|
|
function _singularAdjective($text)
|
|
{
|
|
return $singularText;
|
|
}
|
|
|
|
function _singularSpecialNoun($text)
|
|
{
|
|
return $singularText;
|
|
}
|
|
|
|
function _singularSpecialVerb($text)
|
|
{
|
|
return $singularText;
|
|
}
|
|
|
|
function _singularGeneralVerb($text)
|
|
{
|
|
return $singularText;
|
|
}
|
|
|
|
function _singularSpecialAdjective($text)
|
|
{
|
|
return $singularText;
|
|
}
|
|
|
|
function _enclose($string)
|
|
{
|
|
return '(?:'.$string.')';
|
|
}
|
|
|
|
function _pre($text)
|
|
{
|
|
$inflec =& Inflector::getInstance();
|
|
if (preg_match('/\\A(\\s*)(.+?)(\\s*)\\Z/', $text, $regs))
|
|
{
|
|
if (!empty($regs[1]))
|
|
{
|
|
$inflec->pre = $regs[1];
|
|
}
|
|
|
|
if (!empty($regs[2]))
|
|
{
|
|
$inflec->word = $regs[2];
|
|
}
|
|
|
|
if (!empty($regs[3]))
|
|
{
|
|
$inflec->post = $regs[3];;
|
|
}
|
|
}
|
|
}
|
|
|
|
function _postProcess($orig, $inflected)
|
|
{
|
|
$inflec =& Inflector::getInstance();
|
|
$inflected = preg_replace('/([^|]+)\\|(.+)/', $inflec->classical ? '${2}' : '${1}', $inflected);
|
|
|
|
return $inflected;
|
|
}
|
|
|
|
}
|
|
|
|
echo Inflector::pluralize('rhinoceros');
|
|
?>
|