mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2024-11-15 11:28:25 +00:00
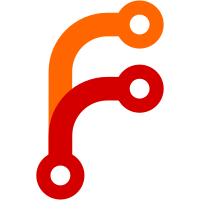
Revision: [1761] Removing old db_acl.sql Revision: [1759] Removed unneeded calls to uses(). Changed basics.php listClasses() no longer using folder class. Starting corrections in DboPostgres class. Adding missing DboPostgres::query(). Added missing doc blocks to AjaxHelper. Fixed undefined keys in FormHelper::generateFields() Reformatted FormHelper::generateFields() adding open and close brackets where needed Revision: [1758] Fixed typo Revision: [1757] Fixed errors found when using PHP 4. Fixed a scaffold error Revision: [1756] Merging changes to model_php4.php Revision: [1755] Fixed scaffolding for the changes made to the model. Fixed Model::isForeignKey(), replaced array_key_exists with in_array, other function was failing Revision: [1754] Committing changes from bundt model to beta. DataSources will not be in the beta release Revision: [1751] Cleaning up a little more in the code. Removing loading of log.php unless it is really needed. Refactored dispatcher to speed up the stripslashes code if it is called Revision: [1748] removing all references to error_messages and deleting the file Revision: [1747] updated more error messages Revision: [1746] removing all error message defines Revision: [1745] added _() method from 1.0 to basics.php only used to return string right now Revision: [1744] Adding fix for ticket #220 Revision: [1743] More work on ErrorHandler class Revision: [1742] Renaming error view for missing database connection Revision: [1741] More work on ErrorHandler class Revision: [1740] More work on error class Revision: [1739] Replacing all $_SERVER variable check with env() in basics.php Revision: [1738] Adding env() to basic Revision: [1737] Updated session to use env() Revision: [1736] Removing ternary operators from Dispatcher Revision: [1735] Per nates request I am rolling back ACL to [1373] Revision: [1734] Removed the IP in the session class this was not very reliable. Added a time setting that generates current time adding the Security::inactiveMins() to the session Removed code that was added to basics.php to replace gethostbyaddr(). Added CAKE_SESSION_STRING define to core.php which is used in the by the Session class to generate a hashed key. Revision: [1733] Moving errors messages to ErrorHandler class. Updating errors view for use with new class. Updating Scaffold to use new class. Updated Dispatcher to use new class. Removing methods from Object class Revision: [1732] Adding ErrorHandler class Revision: [1731] Adding fix for Ticket #223 git-svn-id: https://svn.cakephp.org/repo/trunk/cake@1762 3807eeeb-6ff5-0310-8944-8be069107fe0
369 lines
No EOL
10 KiB
PHP
369 lines
No EOL
10 KiB
PHP
<?php
|
|
/* SVN FILE: $Id$ */
|
|
|
|
/**
|
|
* Dispatcher takes the URL information, parses it for paramters and
|
|
* tells the involved controllers what to do.
|
|
*
|
|
* This is the heart of Cake's operation.
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright (c) 2005, Cake Software Foundation, Inc.
|
|
* 1785 E. Sahara Avenue, Suite 490-204
|
|
* Las Vegas, Nevada 89104
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @copyright Copyright (c) 2005, Cake Software Foundation, Inc.
|
|
* @link http://www.cakefoundation.org/projects/info/cakephp CakePHP Project
|
|
* @package cake
|
|
* @subpackage cake.cake
|
|
* @since CakePHP v 0.2.9
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
|
|
/**
|
|
* List of helpers to include
|
|
*/
|
|
uses('router', DS.'controller'.DS.'controller');
|
|
|
|
/**
|
|
* Dispatcher translates URLs to controller-action-paramter triads.
|
|
*
|
|
* Dispatches the request, creating appropriate models and controllers.
|
|
*
|
|
* @package cake
|
|
* @subpackage cake.cake
|
|
* @since CakePHP v 0.2.9
|
|
*/
|
|
class Dispatcher extends Object
|
|
{
|
|
/**
|
|
* Base URL
|
|
* @var string
|
|
*/
|
|
var $base = false;
|
|
|
|
/**
|
|
* Base URL
|
|
* @var string
|
|
*/
|
|
var $admin = false;
|
|
|
|
/**
|
|
* Base URL
|
|
* @var string
|
|
*/
|
|
var $webservices = null;
|
|
|
|
/**
|
|
* Constructor.
|
|
*/
|
|
function __construct()
|
|
{
|
|
parent::__construct();
|
|
}
|
|
|
|
/**
|
|
* Dispatches and invokes given URL, handing over control to the involved controllers, and then renders the results (if autoRender is set).
|
|
*
|
|
* If no controller of given name can be found, invoke() shows error messages in
|
|
* the form of Missing Controllers information. It does the same with Actions (methods of Controllers are called
|
|
* Actions).
|
|
*
|
|
* @param string $url URL information to work on.
|
|
* @return boolean Success
|
|
*/
|
|
function dispatch($url, $additionalParams=array())
|
|
{
|
|
$params = array_merge($this->parseParams($url), $additionalParams);
|
|
$missingController = false;
|
|
$missingAction = false;
|
|
$missingView = false;
|
|
$privateAction = false;
|
|
|
|
if(defined('CAKE_ADMIN'))
|
|
{
|
|
if(isset($params[CAKE_ADMIN]))
|
|
{
|
|
$this->admin = '/'.CAKE_ADMIN ;
|
|
$url = preg_replace('/'.CAKE_ADMIN.'\//', '', $url);
|
|
if (empty($params['action']))
|
|
{
|
|
$params['action'] = CAKE_ADMIN.'_'.'index';
|
|
}
|
|
else
|
|
{
|
|
$params['action'] = CAKE_ADMIN.'_'.$params['action'];
|
|
}
|
|
}
|
|
}
|
|
|
|
$this->base = $this->baseUrl();
|
|
|
|
if (empty($params['controller']))
|
|
{
|
|
$missingController = true;
|
|
}
|
|
else
|
|
{
|
|
$ctrlName = Inflector::camelize($params['controller']);
|
|
$ctrlClass = $ctrlName.'Controller';
|
|
|
|
if(!class_exists($ctrlClass))
|
|
{
|
|
if (!loadController($ctrlName))
|
|
{
|
|
if(preg_match('/([\\.]+)/',$ctrlName))
|
|
{
|
|
return $this->cakeError('error404',array(array('url' => strtolower($ctrlName),
|
|
'message' => 'Was not found on this server')));
|
|
exit();
|
|
}
|
|
else
|
|
{
|
|
$missingController = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
if ($missingController)
|
|
{
|
|
return $this->cakeError('missingController',array(array('className' => Inflector::camelize($params['controller']."Controller"),
|
|
'webroot' => $this->webroot)));
|
|
}
|
|
else
|
|
{
|
|
$controller =& new $ctrlClass($this);
|
|
}
|
|
|
|
$classMethods = get_class_methods($controller);
|
|
$classVars = get_object_vars($controller);
|
|
|
|
if (empty($params['action']))
|
|
{
|
|
$params['action'] = 'index';
|
|
}
|
|
|
|
if((in_array($params['action'], $classMethods) || in_array(strtolower($params['action']), $classMethods)) && strpos($params['action'], '_', 0) === 0)
|
|
{
|
|
$privateAction = true;
|
|
}
|
|
|
|
if(!in_array($params['action'], $classMethods) && !in_array(strtolower($params['action']), $classMethods))
|
|
{
|
|
$missingAction = true;
|
|
}
|
|
|
|
if(in_array('return', array_keys($params)) && $params['return'] == 1)
|
|
{
|
|
$controller->autoRender = false;
|
|
}
|
|
|
|
$controller->base = $this->base;
|
|
$controller->here = $this->base.'/'.$url;
|
|
$controller->webroot = $this->webroot;
|
|
$controller->params = $params;
|
|
$controller->action = $params['action'];
|
|
$controller->data = empty($params['data'])? null: $params['data'];
|
|
$controller->passed_args = empty($params['pass'])? null: $params['pass'];
|
|
$controller->autoLayout = !$params['bare'];
|
|
$controller->webservices = $params['webservices'];
|
|
|
|
if(!is_null($controller->webservices))
|
|
{
|
|
array_push($controller->components, $controller->webservices);
|
|
array_push($controller->helpers, $controller->webservices);
|
|
}
|
|
|
|
if((in_array('scaffold', array_keys($classVars))) && ($missingAction === true))
|
|
{
|
|
uses(DS.'controller'.DS.'scaffold');
|
|
return new Scaffold($controller, $params);
|
|
}
|
|
|
|
$controller->constructClasses();
|
|
|
|
if ($missingAction)
|
|
{
|
|
return $this->cakeError('missingAction',
|
|
array(array('className' => Inflector::camelize($params['controller']."Controller"),
|
|
'action' => $params['action'],
|
|
'webroot' => $this->webroot)));
|
|
}
|
|
|
|
if ($privateAction)
|
|
{
|
|
return $this->cakeError('privateAction',
|
|
array(array('className' => Inflector::camelize($params['controller']."Controller"),
|
|
'action' => $params['action'],
|
|
'webroot' => $this->webroot)));
|
|
}
|
|
|
|
return $this->_invoke($controller, $params );
|
|
}
|
|
|
|
/**
|
|
* Invokes given controller's render action if autoRender option is set. Otherwise the contents of the operation are returned as a string.
|
|
*
|
|
* @param object $controller
|
|
* @param array $params
|
|
* @return string
|
|
*/
|
|
function _invoke (&$controller, $params )
|
|
{
|
|
$output = call_user_func_array(array(&$controller, $params['action']), empty($params['pass'])? null: $params['pass']);
|
|
if ($controller->autoRender)
|
|
{
|
|
return $controller->render();
|
|
}
|
|
else
|
|
{
|
|
return $output;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Returns array of GET and POST parameters. GET parameters are taken from given URL.
|
|
*
|
|
* @param string $from_url URL to mine for parameter information.
|
|
* @return array Parameters found in POST and GET.
|
|
*/
|
|
function parseParams($from_url)
|
|
{
|
|
// load routes config
|
|
$Route = new Router();
|
|
include CONFIGS.'routes.php';
|
|
$params = $Route->parse ($from_url);
|
|
|
|
if (ini_get('magic_quotes_gpc') == 1)
|
|
{
|
|
if(!empty($_POST))
|
|
{
|
|
if(is_array($_POST))
|
|
{
|
|
$params['form'] = array_map('stripslashes', $_POST);
|
|
}
|
|
else
|
|
{
|
|
$params['form'] = stripcslashes($_POST);
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
$params['form'] = $_POST;
|
|
}
|
|
|
|
if (isset($params['form']['data']))
|
|
{
|
|
$params['data'] = $params['form']['data'];
|
|
}
|
|
|
|
if (isset($_GET))
|
|
{
|
|
if (ini_get('magic_quotes_gpc') == 1)
|
|
{
|
|
if(is_array($_GET))
|
|
{
|
|
$params['url'] = array_map('stripslashes', $_GET);
|
|
}
|
|
else
|
|
{
|
|
$params['url'] = stripcslashes($_GET);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
$params['url'] = $_GET;
|
|
}
|
|
}
|
|
|
|
foreach ($_FILES as $name => $data)
|
|
{
|
|
$params['form'][$name] = $data;
|
|
}
|
|
$params['bare'] = empty($params['ajax'])? (empty($params['bare'])? 0: 1): 1;
|
|
|
|
$params['webservices'] = empty($params['webservices']) ? null : $params['webservices'];
|
|
|
|
return $params;
|
|
}
|
|
|
|
/**
|
|
* Returns a base URL.
|
|
*
|
|
* @return string Base URL
|
|
*/
|
|
function baseUrl()
|
|
{
|
|
$htaccess = null;
|
|
$base = $this->admin;
|
|
$this->webroot = '';
|
|
if (defined('BASE_URL'))
|
|
{
|
|
$base = BASE_URL.$this->admin;
|
|
}
|
|
|
|
$docRoot = env('DOCUMENT_ROOT');
|
|
$scriptName = env('PHP_SELF');
|
|
|
|
// If document root ends with 'webroot', it's probably correctly set
|
|
$r = null;
|
|
if (preg_match('/'.APP_DIR.'\\'.DS.WEBROOT_DIR.'/', $docRoot))
|
|
{
|
|
$this->webroot = '/';
|
|
if (preg_match('/^(.*)\/index\.php$/', $scriptName, $r))
|
|
{
|
|
if(!empty($r[1]))
|
|
{
|
|
return $base.$r[1];
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
if (defined('BASE_URL'))
|
|
{
|
|
$webroot =setUri();
|
|
$htaccess = preg_replace('/(?:'.APP_DIR.'(.*)|index\\.php(.*))/i', '', $webroot).APP_DIR.'/'.WEBROOT_DIR.'/';
|
|
}
|
|
if(APP_DIR === 'app')
|
|
{
|
|
if (preg_match('/^(.*)\\/'.APP_DIR.'\\/'.WEBROOT_DIR.'\\/index\\.php$/', $scriptName, $regs))
|
|
{
|
|
!empty($htaccess)? $this->webroot = $htaccess : $this->webroot = $regs[1].'/';
|
|
return $regs[1];
|
|
}
|
|
else
|
|
{
|
|
!empty($htaccess)? $this->webroot = $htaccess : $this->webroot = '/';
|
|
return $base;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
if (preg_match('/^(.*)\\/'.WEBROOT_DIR.'\\/index\\.php$/', $scriptName, $regs))
|
|
{
|
|
!empty($htaccess)? $this->webroot = $htaccess : $this->webroot = $regs[1].'/';
|
|
return $regs[1];
|
|
}
|
|
else
|
|
{
|
|
!empty($htaccess)? $this->webroot = $htaccess : $this->webroot = '/';
|
|
return $base;
|
|
}
|
|
}
|
|
}
|
|
return $base;
|
|
}
|
|
}
|
|
?>
|