mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2024-11-15 19:38:26 +00:00
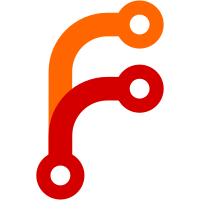
This allows task initialization to be moved out of ShellDispatcher where it does not belong, as tasks are similar to components. Updating parts of TaskCollection, as the Dispatcher is still required to be passed around.
143 lines
No EOL
4 KiB
PHP
143 lines
No EOL
4 KiB
PHP
<?php
|
|
/**
|
|
* TaskCollectionTest file
|
|
*
|
|
* PHP 5
|
|
*
|
|
* CakePHP(tm) : Rapid Development Framework (http://cakephp.org)
|
|
* Copyright 2005-2010, Cake Software Foundation, Inc. (http://cakefoundation.org)
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @copyright Copyright 2005-2010, Cake Software Foundation, Inc. (http://cakefoundation.org)
|
|
* @link http://book.cakephp.org/view/1196/Testing CakePHP(tm) Tests
|
|
* @package cake
|
|
* @subpackage cake.tests.cases.libs
|
|
* @since CakePHP(tm) v 2.0
|
|
* @license MIT License (http://www.opensource.org/licenses/mit-license.php)
|
|
*/
|
|
|
|
App::import('Shell', 'TaskCollection', false);
|
|
App::import('Shell', 'Shell', false);
|
|
|
|
class TaskCollectionTest extends CakeTestCase {
|
|
/**
|
|
* setup
|
|
*
|
|
* @return void
|
|
*/
|
|
function setup() {
|
|
$shell = $this->getMock('Shell', array(), array(), '', false);
|
|
$shell->shellPaths = App::path('shells');
|
|
$dispatcher = $this->getMock('ShellDispatcher', array(), array(), '', false);
|
|
$this->Tasks = new TaskCollection($shell, $dispatcher);
|
|
}
|
|
|
|
/**
|
|
* teardown
|
|
*
|
|
* @return void
|
|
*/
|
|
function teardown() {
|
|
unset($this->Tasks);
|
|
}
|
|
|
|
/**
|
|
* test triggering callbacks on loaded tasks
|
|
*
|
|
* @return void
|
|
*/
|
|
function testLoad() {
|
|
$result = $this->Tasks->load('DbConfig');
|
|
$this->assertType('DbConfigTask', $result);
|
|
$this->assertType('DbConfigTask', $this->Tasks->DbConfig);
|
|
|
|
$result = $this->Tasks->attached();
|
|
$this->assertEquals(array('DbConfig'), $result, 'attached() results are wrong.');
|
|
|
|
$this->assertTrue($this->Tasks->enabled('DbConfig'));
|
|
}
|
|
|
|
/**
|
|
* test load and enable = false
|
|
*
|
|
* @return void
|
|
*/
|
|
function testLoadWithEnableFalse() {
|
|
$result = $this->Tasks->load('DbConfig', array(), false);
|
|
$this->assertType('DbConfigTask', $result);
|
|
$this->assertType('DbConfigTask', $this->Tasks->DbConfig);
|
|
|
|
$this->assertFalse($this->Tasks->enabled('DbConfig'), 'DbConfigTask should be disabled');
|
|
}
|
|
/**
|
|
* test missinghelper exception
|
|
*
|
|
* @expectedException MissingTaskFileException
|
|
* @return void
|
|
*/
|
|
function testLoadMissingTaskFile() {
|
|
$result = $this->Tasks->load('ThisTaskShouldAlwaysBeMissing');
|
|
}
|
|
|
|
/**
|
|
* test loading a plugin helper.
|
|
*
|
|
* @return void
|
|
*/
|
|
function testLoadPluginTask() {
|
|
$dispatcher = $this->getMock('ShellDispatcher', array(), array(), '', false);
|
|
$shell = $this->getMock('Shell', array(), array(), '', false);
|
|
$shell->shellPaths = App::path('shells');
|
|
$shell->shellPaths[] = TEST_CAKE_CORE_INCLUDE_PATH . 'tests' . DS . 'test_app' . DS . 'plugins' . DS . 'test_plugin' . DS . 'vendors' . DS . 'shells' . DS;
|
|
$dispatcher->shellPaths = $shell->shellPaths;
|
|
$this->Tasks = new TaskCollection($shell, $dispatcher);
|
|
|
|
$result = $this->Tasks->load('TestPlugin.OtherTask');
|
|
$this->assertType('OtherTaskTask', $result, 'Task class is wrong.');
|
|
$this->assertType('OtherTaskTask', $this->Tasks->OtherTask, 'Class is wrong');
|
|
}
|
|
|
|
/**
|
|
* test unload()
|
|
*
|
|
* @return void
|
|
*/
|
|
function testUnload() {
|
|
$this->Tasks->load('Extract');
|
|
$this->Tasks->load('DbConfig');
|
|
|
|
$result = $this->Tasks->attached();
|
|
$this->assertEquals(array('Extract', 'DbConfig'), $result, 'loaded tasks is wrong');
|
|
|
|
$this->Tasks->unload('DbConfig');
|
|
$this->assertFalse(isset($this->Tasks->DbConfig));
|
|
$this->assertTrue(isset($this->Tasks->Extract));
|
|
|
|
$result = $this->Tasks->attached();
|
|
$this->assertEquals(array('Extract'), $result, 'loaded tasks is wrong');
|
|
}
|
|
|
|
/**
|
|
* test normalizeObjectArray
|
|
*
|
|
* @return void
|
|
*/
|
|
function testnormalizeObjectArray() {
|
|
$tasks = array(
|
|
'Html',
|
|
'Foo.Bar' => array('one', 'two'),
|
|
'Something',
|
|
'Banana.Apple' => array('foo' => 'bar')
|
|
);
|
|
$result = TaskCollection::normalizeObjectArray($tasks);
|
|
$expected = array(
|
|
'Html' => array('class' => 'Html', 'settings' => array()),
|
|
'Bar' => array('class' => 'Foo.Bar', 'settings' => array('one', 'two')),
|
|
'Something' => array('class' => 'Something', 'settings' => array()),
|
|
'Apple' => array('class' => 'Banana.Apple', 'settings' => array('foo' => 'bar')),
|
|
);
|
|
$this->assertEquals($expected, $result);
|
|
}
|
|
} |