mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2024-11-15 19:38:26 +00:00
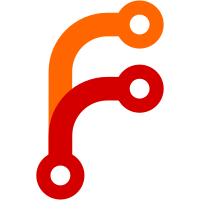
git-svn-id: https://svn.cakephp.org/repo/branches/1.2.x.x@5216 3807eeeb-6ff5-0310-8944-8be069107fe0
203 lines
6.1 KiB
PHP
203 lines
6.1 KiB
PHP
<?php
|
|
/* SVN FILE: $Id$ */
|
|
/**
|
|
* Short description for file.
|
|
*
|
|
* Long description for file
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP(tm) : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright 2005-2007, Cake Software Foundation, Inc.
|
|
* 1785 E. Sahara Avenue, Suite 490-204
|
|
* Las Vegas, Nevada 89104
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @copyright Copyright 2005-2007, Cake Software Foundation, Inc.
|
|
* @link http://www.cakefoundation.org/projects/info/cakephp CakePHP(tm) Project
|
|
* @package cake
|
|
* @subpackage cake.cake.console.libs
|
|
* @since CakePHP(tm) v 1.2.0.5012
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
/**
|
|
* @package cake
|
|
* @subpackage cake.cake.console.libs
|
|
*/
|
|
class ConsoleShell extends Shell {
|
|
var $associations = array('hasOne', 'hasMany', 'belongsTo', 'hasAndBelongsToMany');
|
|
var $badCommandChars = array('$', ';');
|
|
|
|
function initialize() {
|
|
$this->models = @loadModels();
|
|
foreach ($this->models as $model) {
|
|
$class = Inflector::camelize(r('.php', '', $model));
|
|
$this->models[$model] = $class;
|
|
$this->{$class} =& new $class();
|
|
}
|
|
$this->out('Model classes:');
|
|
$this->out('--------------');
|
|
|
|
foreach ($this->models as $model) {
|
|
$this->out(" - {$model}");
|
|
}
|
|
}
|
|
|
|
function main() {
|
|
|
|
while (true) {
|
|
$command = trim($this->in(''));
|
|
|
|
switch($command) {
|
|
case 'help':
|
|
$this->out('Console help:');
|
|
$this->out('-------------');
|
|
$this->out('The interactive console is a tool for testing models before you commit code');
|
|
$this->out('');
|
|
$this->out('To test for results, use the name of your model without a leading $');
|
|
$this->out('e.g. Foo->findAll()');
|
|
$this->out('');
|
|
$this->out('To dynamically set associations, you can do the following:');
|
|
$this->out("\tModelA bind <association> ModelB");
|
|
$this->out("where the supported assocations are hasOne, hasMany, belongsTo, hasAndBelongsToMany");
|
|
$this->out('');
|
|
$this->out('To dynamically remove associations, you can do the following:');
|
|
$this->out("\t ModelA unbind <association> ModelB");
|
|
$this->out("where the supported associations are the same as above");
|
|
break;
|
|
case 'quit':
|
|
case 'exit':
|
|
return true;
|
|
break;
|
|
case 'models':
|
|
$this->out('Model classes:');
|
|
$this->hr();
|
|
foreach ($this->models as $model) {
|
|
$this->out(" - {$model}");
|
|
}
|
|
break;
|
|
case (preg_match("/^(\w+) bind (\w+) (\w+)/", $command, $tmp) == true):
|
|
foreach ($tmp as $data) {
|
|
$data = strip_tags($data);
|
|
$data = str_replace($this->badCommandChars, "", $data);
|
|
}
|
|
|
|
$modelA = $tmp[1];
|
|
$association = $tmp[2];
|
|
$modelB = $tmp[3];
|
|
|
|
if ($this->isValidModel($modelA) && $this->isValidModel($modelB) && in_array($association, $this->associations)) {
|
|
$this->{$modelA}->bindModel(array($association => array($modelB => array('className' => $modelB))), false);
|
|
$this->out("Created $association association between $modelA and $modelB");
|
|
} else {
|
|
$this->out("Please verify you are using valid models and association types");
|
|
}
|
|
break;
|
|
case (preg_match("/^(\w+) unbind (\w+) (\w+)/", $command, $tmp) == true):
|
|
foreach ($tmp as $data) {
|
|
$data = strip_tags($data);
|
|
$data = str_replace($this->badCommandChars, "", $data);
|
|
}
|
|
|
|
$modelA = $tmp[1];
|
|
$association = $tmp[2];
|
|
$modelB = $tmp[3];
|
|
|
|
// Verify that there is actually an association to unbind
|
|
$currentAssociations = $this->{$modelA}->getAssociated();
|
|
$validCurrentAssociation = false;
|
|
|
|
foreach ($currentAssociations as $model => $currentAssociation) {
|
|
if ($model == $modelB && $association == $currentAssociation) {
|
|
$validCurrentAssociation = true;
|
|
}
|
|
}
|
|
|
|
if ($this->isValidModel($modelA) && $this->isValidModel($modelB) && in_array($association, $this->associations) && $validCurrentAssociation) {
|
|
$this->{$modelA}->unbindModel(array($association => array($modelB)));
|
|
$this->out("Removed $association association between $modelA and $modelB");
|
|
} else {
|
|
$this->out("Please verify you are using valid models, valid current association, and valid association types");
|
|
}
|
|
break;
|
|
case (strpos($command, "->find") > 0):
|
|
// Remove any bad info
|
|
$command = strip_tags($command);
|
|
$command = str_replace($this->badCommandChars, "", $command);
|
|
$command = str_replace(";", "", $command);
|
|
|
|
// Do we have a valid model?
|
|
list($modelToCheck, $tmp) = explode('->', $command);
|
|
|
|
if ($this->isValidModel($modelToCheck)) {
|
|
$findCommand = "\$data = \$this->$command;";
|
|
@eval($findCommand);
|
|
|
|
if (is_array($data)) {
|
|
foreach ($data as $idx => $results) {
|
|
if (is_numeric($idx)) { // findAll() output
|
|
foreach ($results as $modelName => $result) {
|
|
$this->out("$modelName");
|
|
|
|
foreach ($result as $field => $value) {
|
|
if (is_array($value)) {
|
|
foreach($value as $field2 => $value2) {
|
|
$this->out("\t$field2: $value2");
|
|
}
|
|
|
|
$this->out("");
|
|
} else {
|
|
$this->out("\t$field: $value");
|
|
}
|
|
}
|
|
}
|
|
} else { // find() output
|
|
$this->out($idx);
|
|
|
|
foreach($results as $field => $value) {
|
|
if (is_array($value)) {
|
|
foreach ($value as $field2 => $value2) {
|
|
$this->out("\t$field2: $value2");
|
|
}
|
|
|
|
$this->out("");
|
|
} else {
|
|
$this->out("\t$field: $value");
|
|
}
|
|
}
|
|
}
|
|
}
|
|
} else {
|
|
$this->out("\nNo result set found");
|
|
}
|
|
} else {
|
|
$this->out("$modelToCheck is not a valid model");
|
|
}
|
|
|
|
break;
|
|
|
|
default:
|
|
$this->out("Invalid command\n");
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
function isValidModel($modelToCheck)
|
|
{
|
|
if (in_array($modelToCheck, $this->models)) {
|
|
return true;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
}
|
|
|
|
|
|
?>
|