mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2024-11-15 11:28:25 +00:00
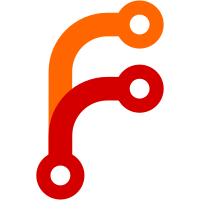
As recommended by the Cookbook: http://book.cakephp.org/2.0/en/development/testing.html#testing-controllers > When testing actions that contain redirect() and other code following the redirect it is generally a good idea to return when redirecting. The reason for this, is that redirect() is mocked in testing, and does not exit like normal. And instead of your code exiting, it will continue to run code following the redirect.
159 lines
5.9 KiB
PHP
159 lines
5.9 KiB
PHP
<?php
|
|
/**
|
|
* Bake Template for Controller action generation.
|
|
*
|
|
* PHP 5
|
|
*
|
|
* CakePHP(tm) : Rapid Development Framework (http://cakephp.org)
|
|
* Copyright (c) Cake Software Foundation, Inc. (http://cakefoundation.org)
|
|
*
|
|
* Licensed under The MIT License
|
|
* For full copyright and license information, please see the LICENSE.txt
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @copyright Copyright (c) Cake Software Foundation, Inc. (http://cakefoundation.org)
|
|
* @link http://cakephp.org CakePHP(tm) Project
|
|
* @package Cake.Console.Templates.default.actions
|
|
* @since CakePHP(tm) v 1.3
|
|
* @license http://www.opensource.org/licenses/mit-license.php MIT License
|
|
*/
|
|
?>
|
|
|
|
/**
|
|
* <?php echo $admin ?>index method
|
|
*
|
|
* @return void
|
|
*/
|
|
public function <?php echo $admin ?>index() {
|
|
$this-><?php echo $currentModelName ?>->recursive = 0;
|
|
$this->set('<?php echo $pluralName ?>', $this->Paginator->paginate());
|
|
}
|
|
|
|
/**
|
|
* <?php echo $admin ?>view method
|
|
*
|
|
* @throws NotFoundException
|
|
* @param string $id
|
|
* @return void
|
|
*/
|
|
public function <?php echo $admin ?>view($id = null) {
|
|
if (!$this-><?php echo $currentModelName; ?>->exists($id)) {
|
|
throw new NotFoundException(__('Invalid <?php echo strtolower($singularHumanName); ?>'));
|
|
}
|
|
$options = array('conditions' => array('<?php echo $currentModelName; ?>.' . $this-><?php echo $currentModelName; ?>->primaryKey => $id));
|
|
$this->set('<?php echo $singularName; ?>', $this-><?php echo $currentModelName; ?>->find('first', $options));
|
|
}
|
|
|
|
<?php $compact = array(); ?>
|
|
/**
|
|
* <?php echo $admin ?>add method
|
|
*
|
|
* @return void
|
|
*/
|
|
public function <?php echo $admin ?>add() {
|
|
if ($this->request->is('post')) {
|
|
$this-><?php echo $currentModelName; ?>->create();
|
|
if ($this-><?php echo $currentModelName; ?>->save($this->request->data)) {
|
|
<?php if ($wannaUseSession): ?>
|
|
$this->Session->setFlash(__('The <?php echo strtolower($singularHumanName); ?> has been saved'));
|
|
return $this->redirect(array('action' => 'index'));
|
|
<?php else: ?>
|
|
$this->flash(__('<?php echo ucfirst(strtolower($currentModelName)); ?> saved.'), array('action' => 'index'));
|
|
<?php endif; ?>
|
|
} else {
|
|
<?php if ($wannaUseSession): ?>
|
|
$this->Session->setFlash(__('The <?php echo strtolower($singularHumanName); ?> could not be saved. Please, try again.'));
|
|
<?php endif; ?>
|
|
}
|
|
}
|
|
<?php
|
|
foreach (array('belongsTo', 'hasAndBelongsToMany') as $assoc):
|
|
foreach ($modelObj->{$assoc} as $associationName => $relation):
|
|
if (!empty($associationName)):
|
|
$otherModelName = $this->_modelName($associationName);
|
|
$otherPluralName = $this->_pluralName($associationName);
|
|
echo "\t\t\${$otherPluralName} = \$this->{$currentModelName}->{$otherModelName}->find('list');\n";
|
|
$compact[] = "'{$otherPluralName}'";
|
|
endif;
|
|
endforeach;
|
|
endforeach;
|
|
if (!empty($compact)):
|
|
echo "\t\t\$this->set(compact(".join(', ', $compact)."));\n";
|
|
endif;
|
|
?>
|
|
}
|
|
|
|
<?php $compact = array(); ?>
|
|
/**
|
|
* <?php echo $admin ?>edit method
|
|
*
|
|
* @throws NotFoundException
|
|
* @param string $id
|
|
* @return void
|
|
*/
|
|
public function <?php echo $admin; ?>edit($id = null) {
|
|
if (!$this-><?php echo $currentModelName; ?>->exists($id)) {
|
|
throw new NotFoundException(__('Invalid <?php echo strtolower($singularHumanName); ?>'));
|
|
}
|
|
if ($this->request->is('post') || $this->request->is('put')) {
|
|
if ($this-><?php echo $currentModelName; ?>->save($this->request->data)) {
|
|
<?php if ($wannaUseSession): ?>
|
|
$this->Session->setFlash(__('The <?php echo strtolower($singularHumanName); ?> has been saved'));
|
|
return $this->redirect(array('action' => 'index'));
|
|
<?php else: ?>
|
|
$this->flash(__('The <?php echo strtolower($singularHumanName); ?> has been saved.'), array('action' => 'index'));
|
|
<?php endif; ?>
|
|
} else {
|
|
<?php if ($wannaUseSession): ?>
|
|
$this->Session->setFlash(__('The <?php echo strtolower($singularHumanName); ?> could not be saved. Please, try again.'));
|
|
<?php endif; ?>
|
|
}
|
|
} else {
|
|
$options = array('conditions' => array('<?php echo $currentModelName; ?>.' . $this-><?php echo $currentModelName; ?>->primaryKey => $id));
|
|
$this->request->data = $this-><?php echo $currentModelName; ?>->find('first', $options);
|
|
}
|
|
<?php
|
|
foreach (array('belongsTo', 'hasAndBelongsToMany') as $assoc):
|
|
foreach ($modelObj->{$assoc} as $associationName => $relation):
|
|
if (!empty($associationName)):
|
|
$otherModelName = $this->_modelName($associationName);
|
|
$otherPluralName = $this->_pluralName($associationName);
|
|
echo "\t\t\${$otherPluralName} = \$this->{$currentModelName}->{$otherModelName}->find('list');\n";
|
|
$compact[] = "'{$otherPluralName}'";
|
|
endif;
|
|
endforeach;
|
|
endforeach;
|
|
if (!empty($compact)):
|
|
echo "\t\t\$this->set(compact(".join(', ', $compact)."));\n";
|
|
endif;
|
|
?>
|
|
}
|
|
|
|
/**
|
|
* <?php echo $admin ?>delete method
|
|
*
|
|
* @throws NotFoundException
|
|
* @param string $id
|
|
* @return void
|
|
*/
|
|
public function <?php echo $admin; ?>delete($id = null) {
|
|
$this-><?php echo $currentModelName; ?>->id = $id;
|
|
if (!$this-><?php echo $currentModelName; ?>->exists()) {
|
|
throw new NotFoundException(__('Invalid <?php echo strtolower($singularHumanName); ?>'));
|
|
}
|
|
$this->request->onlyAllow('post', 'delete');
|
|
if ($this-><?php echo $currentModelName; ?>->delete()) {
|
|
<?php if ($wannaUseSession): ?>
|
|
$this->Session->setFlash(__('<?php echo ucfirst(strtolower($singularHumanName)); ?> deleted'));
|
|
return $this->redirect(array('action' => 'index'));
|
|
<?php else: ?>
|
|
$this->flash(__('<?php echo ucfirst(strtolower($singularHumanName)); ?> deleted'), array('action' => 'index'));
|
|
<?php endif; ?>
|
|
}
|
|
<?php if ($wannaUseSession): ?>
|
|
$this->Session->setFlash(__('<?php echo ucfirst(strtolower($singularHumanName)); ?> was not deleted'));
|
|
<?php else: ?>
|
|
$this->flash(__('<?php echo ucfirst(strtolower($singularHumanName)); ?> was not deleted'), array('action' => 'index'));
|
|
<?php endif; ?>
|
|
return $this->redirect(array('action' => 'index'));
|
|
}
|