mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2024-11-15 11:28:25 +00:00
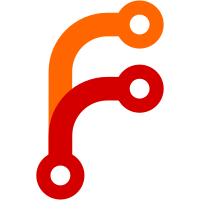
git-svn-id: https://svn.cakephp.org/repo/trunk/cake@1596 3807eeeb-6ff5-0310-8944-8be069107fe0
186 lines
No EOL
6.6 KiB
Text
186 lines
No EOL
6.6 KiB
Text
<?php
|
|
/* SVN FILE: $Id$ */
|
|
|
|
/**
|
|
* Base controller class.
|
|
*
|
|
* PHP versions 4 and 5
|
|
*
|
|
* CakePHP : Rapid Development Framework <http://www.cakephp.org/>
|
|
* Copyright (c) 2005, Cake Software Foundation, Inc.
|
|
* 1785 E. Sahara Avenue, Suite 490-204
|
|
* Las Vegas, Nevada 89104
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @filesource
|
|
* @copyright Copyright (c) 2005, Cake Software Foundation, Inc.
|
|
* @link http://www.cakefoundation.org/projects/info/cakephp CakePHP Project
|
|
* @package cake
|
|
* @subpackage cake.cake.libs.controller.templates.scaffolds
|
|
* @since CakePHP v 0.10.0.1076
|
|
* @version $Revision$
|
|
* @modifiedby $LastChangedBy$
|
|
* @lastmodified $Date$
|
|
* @license http://www.opensource.org/licenses/mit-license.php The MIT License
|
|
*/
|
|
?>
|
|
|
|
<?php
|
|
$modelName = Inflector::singularize($this->name);
|
|
$modelKey = Inflector::underscore($modelName);
|
|
$registry =& ClassRegistry::getInstance();
|
|
$objModel = $registry->getObject($modelKey);
|
|
|
|
?>
|
|
<h1>Show <?php echo Inflector::humanize($modelName)?></h1>
|
|
|
|
<dl>
|
|
<?php foreach($fieldNames as $field => $value)
|
|
{
|
|
echo "<dt>".$value['prompt']."</dt>";
|
|
if(isset($value['foreignKey']))
|
|
{
|
|
$alias = array_search($value['table'],$objModel->alias);
|
|
$otherModelObject = $registry->getObject(Inflector::underscore($objModel->tableToModel[$value['table']]));
|
|
$displayField = $otherModelObject->getDisplayField();
|
|
$displayText = $data[$alias][$displayField];
|
|
|
|
if(!empty($data[$objModel->tableToModel[$objModel->table]][$field]))
|
|
{
|
|
echo "<dd>".$html->linkTo($displayText, '/'.Inflector::underscore($value['controller']).'/show/'
|
|
.$data[$objModel->tableToModel[$objModel->table]][$field] )."</dd>";
|
|
}
|
|
else
|
|
{
|
|
echo "<dd> </dd>";
|
|
}
|
|
}
|
|
else
|
|
{
|
|
// this is just a plain old field.
|
|
if( !empty($data[$objModel->tableToModel[$objModel->table]][$field]))
|
|
{
|
|
echo "<dd>".$data[$objModel->tableToModel[$objModel->table]][$field]."</dd>";
|
|
}
|
|
else
|
|
{
|
|
echo "<dd> </dd>";
|
|
}
|
|
}
|
|
}
|
|
?>
|
|
|
|
</dl>
|
|
<ul class='actions'>
|
|
<?php
|
|
echo "<li>".$html->linkTo('Edit '.Inflector::humanize($objModel->name), '/'.$this->viewPath.'/edit/'.$data[$objModel->tableToModel[$objModel->table]][$this->controller->{$modelName}->primaryKey])."</li>";
|
|
echo "<li>".$html->linkTo('Delete '.Inflector::humanize($objModel->name), '/'.$this->viewPath.'/destroy/'.$data[$objModel->tableToModel[$objModel->table]][$this->controller->{$modelName}->primaryKey])."</li>";
|
|
echo "<li>".$html->linkTo('List '.Inflector::humanize($objModel->name), '/'.$this->viewPath.'/index')."</li>";
|
|
echo "<li>".$html->linkTo('New '.Inflector::humanize($objModel->name), '/'.$this->viewPath.'/add')."</li>";
|
|
foreach( $fieldNames as $field => $value ) {
|
|
if( isset( $value['foreignKey'] ) )
|
|
{
|
|
echo "<li>".$html->linkTo( "View ".Inflector::humanize($value['controller']), "/".Inflector::underscore($value['controller'])."/show/".$data[$alias][$otherModelObject->primaryKey] )."</li>";
|
|
}
|
|
}
|
|
?>
|
|
</ul>
|
|
|
|
<!--hasOne relationships -->
|
|
<?php
|
|
foreach ($objModel->_oneToOne as $relation)
|
|
{
|
|
list($association, $model, $value) = $relation;
|
|
$otherModelName = $objModel->tableToModel[$model];
|
|
$controller = Inflector::pluralize($model);
|
|
|
|
echo "<div class='related'><H2>Related ".Inflector::humanize($association)."</H2>";
|
|
echo "<dl>";
|
|
if( isset($data[$association]) && is_array($data[$association]) )
|
|
{
|
|
foreach( $data[$association] as $field=>$value )
|
|
{
|
|
echo "<dt>".Inflector::humanize($field)."</dt>";
|
|
if( !empty($value) )
|
|
{
|
|
echo "<dd>".$value."</dd>";
|
|
} else {
|
|
echo "<dd> </dd>";
|
|
}
|
|
}
|
|
|
|
}
|
|
echo "</dl>";
|
|
echo "<ul class='actions'><li>".$html->linkTo('Edit '.Inflector::humanize($association),"/".Inflector::underscore($controller)."/edit/{$data[$association][$otherModelObject->primaryKey]}")."</li></ul></div>";
|
|
}
|
|
?>
|
|
|
|
<!-- HAS MANY AND HASANDBELONGSTOMANY -->
|
|
<?php
|
|
$relations = array();
|
|
foreach( $objModel->_oneToMany as $relation )
|
|
{
|
|
$relations[] = $relation;
|
|
} // end loop through onetomany relations.
|
|
|
|
foreach( $objModel->_manyToMany as $relation )
|
|
{
|
|
$relations[] = $relation;
|
|
} // end loop through manytomany relations.
|
|
|
|
foreach( $relations as $relation )
|
|
{
|
|
list($association, $model, $value) = $relation;
|
|
$count = 0;
|
|
$otherModelName = Inflector::singularize($model);
|
|
$controller = Inflector::pluralize($model);
|
|
|
|
echo "<div class='related'><H2>Related ".Inflector::humanize(Inflector::pluralize($association))."</H2>";
|
|
if( isset($data[$association]) && is_array($data[$association]) )
|
|
{
|
|
?>
|
|
|
|
<table class="inav" cellspacing="0">
|
|
<tr>
|
|
<?php // Loop through and create the header row.
|
|
// find a row that matches this title.
|
|
$bFound = false;
|
|
foreach( $data[$association][0] as $column=>$value ) {
|
|
echo "<th>".Inflector::humanize($column)."</th>";
|
|
}
|
|
?>
|
|
<th>Actions</th>
|
|
</tr>
|
|
<?php
|
|
// now find all matching rows
|
|
foreach( $data[$association] as $row )
|
|
{
|
|
echo "<tr>";
|
|
foreach( $row as $column=>$value )
|
|
{
|
|
echo "<td>".$value."</td>";
|
|
}
|
|
?>
|
|
<td class="listactions"><?php echo $html->linkTo('View',"/".Inflector::underscore($controller)."/show/{$row[$this->controller->{$modelName}->{$association}->primaryKey]}/")?>
|
|
<?php echo $html->linkTo('Edit',"/".Inflector::underscore($controller)."/edit/{$row[$this->controller->{$modelName}->{$association}->primaryKey]}/")?>
|
|
<?php echo $html->linkTo('Delete',"/".Inflector::underscore($controller)."/destroy/{$row[$this->controller->{$modelName}->{$association}->primaryKey]}/")?>
|
|
</td>
|
|
<?php
|
|
echo "</tr>";
|
|
}
|
|
}
|
|
?>
|
|
|
|
</table>
|
|
<ul class="actions">
|
|
<?php
|
|
// add a link to create a new relation.
|
|
|
|
echo "<li>".$html->linkTo('New '.Inflector::humanize($association),"/".Inflector::underscore($controller)."/add/")."</li>";
|
|
?>
|
|
</ul></div>
|
|
|
|
<?php } // end loop through relations
|
|
?> |