mirror of
https://github.com/kamilwylegala/cakephp2-php8.git
synced 2025-04-17 06:23:02 +00:00
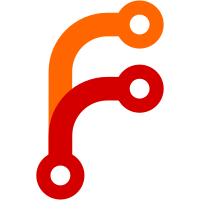
The option to sort has been removed and the list of shells is now sorted and grouped by plugin and then by command. Core and app shells are always listed last.
170 lines
4.8 KiB
PHP
170 lines
4.8 KiB
PHP
<?php
|
|
/**
|
|
* CakePHP(tm) : Rapid Development Framework (http://cakephp.org)
|
|
* Copyright 2005-2012, Cake Software Foundation, Inc. (http://cakefoundation.org)
|
|
*
|
|
* Licensed under The MIT License
|
|
* Redistributions of files must retain the above copyright notice.
|
|
*
|
|
* @copyright Copyright 2005-2012, Cake Software Foundation, Inc. (http://cakefoundation.org)
|
|
* @link http://cakephp.org CakePHP Project
|
|
* @package Cake.Console.Command
|
|
* @since CakePHP v 2.0
|
|
* @license MIT License (http://www.opensource.org/licenses/mit-license.php)
|
|
*/
|
|
|
|
App::uses('AppShell', 'Console/Command');
|
|
App::uses('Inflector', 'Utility');
|
|
|
|
/**
|
|
* Shows a list of commands available from the console.
|
|
*
|
|
* @package Cake.Console.Command
|
|
*/
|
|
class CommandListShell extends AppShell {
|
|
|
|
/**
|
|
* startup
|
|
*
|
|
* @return void
|
|
*/
|
|
public function startup() {
|
|
if (empty($this->params['xml'])) {
|
|
parent::startup();
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Main function Prints out the list of shells.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function main() {
|
|
if (empty($this->params['xml'])) {
|
|
$this->out(__d('cake_console', "<info>Current Paths:</info>"), 2);
|
|
$this->out(" -app: " . APP_DIR);
|
|
$this->out(" -working: " . rtrim(APP, DS));
|
|
$this->out(" -root: " . rtrim(ROOT, DS));
|
|
$this->out(" -core: " . rtrim(CORE_PATH, DS));
|
|
$this->out("");
|
|
$this->out(__d('cake_console', "<info>Changing Paths:</info>"), 2);
|
|
$this->out(__d('cake_console', "Your working path should be the same as your application path to change your path use the '-app' param."));
|
|
$this->out(__d('cake_console', "Example: -app relative/path/to/myapp or -app /absolute/path/to/myapp"), 2);
|
|
|
|
$this->out(__d('cake_console', "<info>Available Shells:</info>"), 2);
|
|
}
|
|
|
|
$shellList = $this->_getShellList();
|
|
if (empty($shellList)) {
|
|
return;
|
|
}
|
|
|
|
if (empty($this->params['xml'])) {
|
|
$this->_asText($shellList);
|
|
} else {
|
|
$this->_asXml($shellList);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Gets the shell command listing.
|
|
*
|
|
* @return array
|
|
*/
|
|
protected function _getShellList() {
|
|
$skipFiles = array('AppShell');
|
|
|
|
$plugins = CakePlugin::loaded();
|
|
$shellList = array_fill_keys($plugins, null) + array('CORE' => null, 'app' => null);
|
|
|
|
$corePath = App::core('Console/Command');
|
|
$shells = App::objects('file', $corePath[0]);
|
|
$shells = array_diff($shells, $skipFiles);
|
|
$this->_appendShells('CORE', $shells, $shellList);
|
|
|
|
$appShells = App::objects('Console/Command', null, false);
|
|
$appShells = array_diff($appShells, $shells, $skipFiles);
|
|
$this->_appendShells('app', $appShells, $shellList);
|
|
|
|
foreach ($plugins as $plugin) {
|
|
$pluginShells = App::objects($plugin . '.Console/Command');
|
|
$this->_appendShells($plugin, $pluginShells, $shellList);
|
|
}
|
|
|
|
return array_filter($shellList);
|
|
}
|
|
|
|
/**
|
|
* Scan the provided paths for shells, and append them into $shellList
|
|
*
|
|
* @param string $type
|
|
* @param array $shells
|
|
* @param array $shellList
|
|
* @return array
|
|
*/
|
|
protected function _appendShells($type, $shells, &$shellList) {
|
|
foreach ($shells as $shell) {
|
|
$shellList[$type][] = Inflector::underscore(str_replace('Shell', '', $shell));
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Output text.
|
|
*
|
|
* @param array $shellList
|
|
* @return void
|
|
*/
|
|
protected function _asText($shellList) {
|
|
foreach ($shellList as $plugin => $commands) {
|
|
sort($commands);
|
|
$this->out(sprintf('[<info>%s</info>] %s', $plugin, implode(', ', $commands)));
|
|
$this->out();
|
|
}
|
|
|
|
$this->out(__d('cake_console', "To run an app or core command, type <info>cake shell_name [args]</info>"));
|
|
$this->out(__d('cake_console', "To run a plugin command, type <info>cake Plugin.shell_name [args]</info>"));
|
|
$this->out(__d('cake_console', "To get help on a specific command, type <info>cake shell_name --help</info>"), 2);
|
|
}
|
|
|
|
/**
|
|
* Output as XML
|
|
*
|
|
* @param array $shellList
|
|
* @return void
|
|
*/
|
|
protected function _asXml($shellList) {
|
|
$plugins = CakePlugin::loaded();
|
|
$shells = new SimpleXmlElement('<shells></shells>');
|
|
foreach ($shellList as $plugin => $commands) {
|
|
foreach ($commands as $command) {
|
|
$callable = $command;
|
|
if (in_array($plugin, $plugins)) {
|
|
$callable = Inflector::camelize($plugin) . '.' . $command;
|
|
}
|
|
|
|
$shell = $shells->addChild('shell');
|
|
$shell->addAttribute('name', $command);
|
|
$shell->addAttribute('call_as', $callable);
|
|
$shell->addAttribute('provider', $plugin);
|
|
$shell->addAttribute('help', $callable . ' -h');
|
|
}
|
|
}
|
|
$this->stdout->outputAs(ConsoleOutput::RAW);
|
|
$this->out($shells->saveXml());
|
|
}
|
|
|
|
/**
|
|
* get the option parser
|
|
*
|
|
* @return void
|
|
*/
|
|
public function getOptionParser() {
|
|
$parser = parent::getOptionParser();
|
|
return $parser->description(__d('cake_console', 'Get the list of available shells for this CakePHP application.'))
|
|
->addOption('xml', array(
|
|
'help' => __d('cake_console', 'Get the listing as XML.'),
|
|
'boolean' => true
|
|
));
|
|
}
|
|
|
|
}
|